indianajonesilm@Posted: Wed Sep 22, 2004 2:40 am : Updated on October 3, 2004
Creating a simple cinematic with a moving camera.
Hello, this is my first tutorial. I'm a newbie, so if you find any mistakes please let me know.
Step 1.
Lets start by making a small room and add an "info_player_start" and a light.
Step 2.
Add a "func_cameraview".
Step 3.
Next make a NURB spline path for the camera to follow and name it "camera_spline".
Read Rayne's excellent NURBS tutorial here:
http://www.doom3world.org/phpbb2/viewtopic.php?t=5123
Instead of moving a light around the room, we will be moving the camera along this path.
Step 4.
Create a "func_mover" and name it "anchor". You must add a Key of cinematic" with a Value of "1". This is very important if you want the camera to move. I also add the Key "solid" with the Value of "0" so the camera can pass through stuff. You should also texture this func_mover with the "common\nodraw" texture.

Step 5.
Next we need to bind the func_camera view to the func_mover. Select the "func_cameraview" and add the Key "bind" with a Value of "anchor" in the entity inspectors window.
Step 6.
Add a "trigger_once" and give it a Key "call" with a Value of "camera_script". This is the trigger that will call the script we make in the next step.
Step 7.
That's it, now we need to write a script to switch the view to the func_cameraview and start it moving along the spline. Open up notepad and type the following:
Code:
void start_camera_spline()
{
$anchor.time(10); //How many seconds it will take to follow the spline from start to finish
$anchor.accelTime(1); //How long it takes to get up to speed
$anchor.decelTime(1); //How long it takes to decelerate
$anchor.startSpline($camera_spline); //Start the func_mover "anchor" moving along the spline
}
void camera_script()
{
sys.setCamera($func_cameraview_1); //Set the view to the camera
start_camera_spline(); //calls the script above
sys.wait(10); //The time to wait before switching view back to normal
sys.firstPerson(); //Turn off the camera and switch back to normal view
}
Step 8.
Save the script above as "your map name.script" and save it in the same folder as your map. For example if your map is called "cave.map" then name your script "cave.script".
Step 9.
Compile your map and start it up! As soon as you touch the "trigger_once" the view will switch to the camera and follow the spline path. If you wanted to make an intro cinematic before your map starts then either place the trigger on your info_player_start or just connect(Ctrl-K) your info_player_start with a "trigger_relay" that calls the script.
Step 10 (and other cool stuff).
Remember to add the
Key "cinematic" with the Value of "1" to all your func_mover's, func_rotator's, characters, and other entities that you want to see move in your cinematic.
Be sure to add the key to all lights that are triggered in your cinematic also. Any entity that is used during the cinematic, has to have this key set. (thanks Docgalaad). You can add special camera tricks to your cinematic by playing with the FOV (frame of view) to get cool warp effects. You can have many different cameras and just switch the view to another one whenever you want by triggering that camera with script or a trigger. If this tut has helped you out and you want to thank me, just mention me in the end credits of your map!


Here is an example of a simple cinematic which I made using the editor.
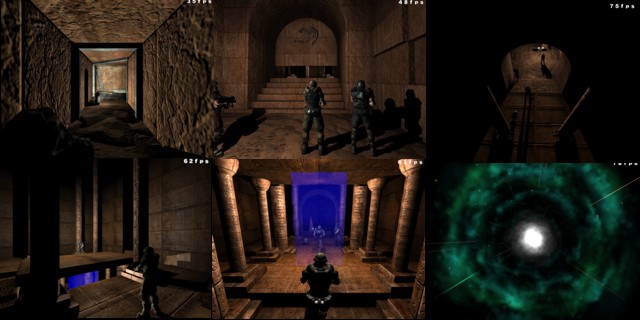
Download the pk4 here:
http://webpages.charter.net/aldanafx/stuff/cinematic_example.zipPlace the "cinematic.pk4" in your base folder and type "map cinematic" in the console to start it.
Update October 3, 2004How to aim the moving camera.(Thanks to rich_is_bored and MBolus for the help with the script)
Step 1.
First we need something for the camera to aim at, so we will add a "target_null" to the map. Place the target_null anywhere you want, and the camera will continuously aim at it while it travels along the spline. The target_null doesn't have to be in a fixed position, it can be binded to a mover and move along it's own spline if you wish.
Step 2.
Next we need to add a "target_setkeyval" to the map. A target_setkeyval is used to set or change Key's and Values of a targeted entity. In this case we will be using this target_setkeyval to add a Key\Val pair to the func_camera view. Now connect (Ctrl-K) the "target_setkeyval" to the "func_cameraview".
Step 3.
Select the "target_setkeyval" and give it a Key of "keyval" and a value of "target;target_null_1". It should look likethe following picture:

Step 4.
Now we need to add some additional script to tell the camera to continually aim at the target_null while it moves along the spline. Here is the previous script from above with the new aiming script added in:
Code:
void start_camera_spline()
{
$anchor.time(20);
$anchor.accelTime(1);
$anchor.decelTime(1);
$anchor.disableSplineAngles(); //You need to add this when aiming a moving camera.
$anchor.startSpline($camera_spline);
}
//This is the new aiming script:
void update_camera()
{
sys.threadname("aim_loop"); // Gives this thread the name "aim_loop" so it can be killed later.
while (1)
{
//This triggers the target_setkeyvall which in turn adds a key/val pair to the func_cameraview:
sys.trigger($target_setkeyval_1);
sys.waitFrame(); //This waits for the next frame before continuing
}
}
void camera_script()
{
sys.setCamera($func_cameraview_1);
start_camera_spline();
thread update_camera(); // This calls the function update_camera as a thread so it
// runs in the background.
sys.waitFor($anchor); // This halts execution until anchor finishes it's movement.
sys.killthread("aim_loop"); // This kills the thread "aim_loop"
sys.firstPerson(); //Turn off the camera and switch back to normal
}
Step 5.
As you see above, it is nessecary to use the "disableSplineAngles" command in your script when tracking something with the moving camera.
That's it, your camera should now aim at the target_null while it moves along the spline.
rich_is_bored@Posted: Wed Sep 22, 2004 3:06 am : Ah!
* slaps forehead *
I tried this exact thing before. The only difference was i didn't know about this cinematic key/value pair.
Thanks alot!

I'll try this out soon and verify if you have any corrections to make.
MBolus@Posted: Wed Sep 22, 2004 3:43 am : I have also been using this type of thing, including around areas such as hallways with teleporters, and found it to really enhance a level. Fov of 140 gives a pretty wide indoors view, compared with 90.
I would add a few minor points. There are a few additional useful keys. For example, you can specify whether an entity will hide after its anims, or whether it will stay to potentially attack, and there is a key you could use for the func_cameraview to indicate whether non-cinematic entities with enemies will be seen. Also, if you preferred you could use triggers to start and stop the new camera view.
rich_is_bored@Posted: Thu Sep 23, 2004 4:49 am : I've just tested it out and it works great.

Now the only thing left is to figure out a way to aim the camera at a target while it moves on the spline.
Great work though.

abigserve@Posted: Thu Sep 23, 2004 5:53 am : let us know how that turns out rich

Rayne@Posted: Thu Sep 23, 2004 7:32 am : Oh bè, you have to connect the func_cameraview's to a target_null (when you connect them, the cam view in the editor switch from the cameraview entity).. so you have only to bind and move even the target_null

rich_is_bored@Posted: Thu Sep 23, 2004 8:11 pm : Your right, targeting the camera_view to a target_null aims the camera.
The problem is that while the camera is initially aimed at the target_null when the map loads, it doesn't follow the null should the camera or null's position change.
indianajonesilm@Posted: Thu Sep 23, 2004 8:57 pm : Quote:
Now the only thing left is to figure out a way to aim the camera at a target while it moves on the spline.
I'm working on it.

I hope there is a way to do it. I added a little example of a cinematic for everyone. (you're in the credits

) I hope my free isp webspace doesn't exceed it's bandwidth limit.
rich_is_bored@Posted: Thu Sep 23, 2004 9:40 pm : That was impressive.

I hope you have the bandwidth to handle all the traffic you're going to get.
elroacho@Posted: Fri Sep 24, 2004 1:41 am : works like a charm...
a question tho, i want to pause the camera at the end of the spline for a few seconds while a big platform(func_door) moves down. i trigger the platform and the speakers for the sound of the moving plat a few seconds into the cinimatic. i can hear the souds of the plat but the func_door dosen't move until the cinimatic has completed and i can move again.
is their something special i have to do to trigger it to play in a cinimatic? if i trigger it with out the camera the func_door and the sound start at the same time like it's supposed to.
1 more thing, lets say you have a trigger to the cinimatic right before a door. then you put in a "recycling2_mancintro_player" to show the charector walking in the cinimatic. how to you make it so that when the cinimatic finishes, the player starts at a different spot then where they triggered it? like the player triggeres cinimatic from the beginning postition of "recycling2_mancintro_player" and when it finishes, i want the player to be at the end postition of "recycling2_mancintro_player".
help please, i'm tring real hard

indianajonesilm@Posted: Fri Sep 24, 2004 2:11 am : Quote:
is their something special i have to do to trigger it to play in a cinimatic?
You have to give the door a Key of "cinematic" with a Value of "1" in the entities inspector window in oder to see it move in your cinematic.
Quote:
how to you make it so that when the cinimatic finishes, the player starts at a different spot then where they triggered it?
Try using a "info_player_teleport". Make a Trigger in the start location. Ctrl-K the trigger with the info_player_teleport. When the player touches the tigger, (you can give it the Key "visualView" with the Value "Camera Name") it will switch views to that camera for your cinematic. (Check the usage of the different Key's in the entities inspector window). After the cinematic is over, your player will be in the location of the info_player_teleport.
That might work.
elroacho@Posted: Fri Sep 24, 2004 7:16 am : awesome, worked perfectly
thanx indianajonesilm
abigserve@Posted: Fri Sep 24, 2004 8:33 am : 
not working 4 me....i followed the tut exactly as well...
Oneofthe8devilz@Posted: Fri Sep 24, 2004 9:18 am : this stuff is amazing !!!!
I think this method combined with the animation export tool from "der_ton" are the ideal fundament for making professional machinima movies.
here is an example of what you can do with the tool
http://www.doom3world.org/phpbb2/viewtopic.php?t=5539
So, for the difficult parts like going up the stairs one could remove the stiffness of the models by creating an md5anim.......
Docgalaad@Posted: Sat Sep 25, 2004 2:47 am : OMG I LOST 6 HOURS TO DEBUG A FUNCTION
You have to be
EXTREMLY CAREFUL about the
cinematic key when you wish to move objects in front of a camera !
I didnt know this hidden key/value pair. And to learn how to use a camera, i looked into one used in the game. Unfortunatly, this one didnt make objects to move in the scenery.
I did a function moving one object, and activating a light to have a better view of what's happening. Once the cinematic switch back to first person view, i set the light off again.
My script was perfect until i test it. The script entered in a weird loop, and the camera made the script to never terminate. The game was stuck in the cinematic. I scanned every line and did a ton of modification. Until i look into THIS THREAD on the forum. Worse, i knew this post, i already read it 3 days ago. But i completly passed through the cinematic key issue.
So, if you have this trouble, yes you, i expect you will search for those keyword on the forum:
CAMERA FUNC_MOVER CINEMATIC LIGHT
Why light ? Cause the light on in my cinematic was a part of it, somehow, and i had to add the key/value cinematic/1 on the light, to get out of my cinematic and make the whole script running properly.
Any entity that is used during the cinematic, has to have this key set.
Not only the moving objects. I hope it's clear cause i'm a bit upset about the lost time


Cheers.
wheelbarrow@Posted: Sat Sep 25, 2004 3:02 am : I have a cinematic with 8 lights, 2 of them flashing, and 7 func_emitters, and they all work fine without the 'cinematic' key/value. The only thing with this key is an opening door....
Docgalaad@Posted: Sat Sep 25, 2004 3:04 am : I trust you. But removing the key on the light and my cinematic is stuck forever. The map works perfectly with the key set.
Do you trigger your lights and emitters during the cinematic ?
Cause i do on my part. Maybe...
xflame@Posted: Sun Sep 26, 2004 11:50 pm : I had a strange problem with this, when I ran my file, I got the error:
CANNOT BIND TO ITSELF!
wheelbarrow@Posted: Mon Sep 27, 2004 12:45 am : No, I don't trigger anything but the door during the cinematic. As for the error message above, just like it says, you've tried to bind something to itself. I did this myself by accident a while ago. Just go thru your entities, and make sure you're not binding the mover to itself. Easy mistake, easy to fix

indianajonesilm@Posted: Mon Sep 27, 2004 1:46 am : Quote:
abigserve: not working 4 me....i followed the tut exactly as well...
Sorry abigserve, I'm new to writing tutorials and I'm still figuring out this editor stuff. I've only been using it for a few weeks. I apologize for my inability to write a decent tutorial. Maybe I can help figure out the problem you're having if you give me a little more info. Did the view switch to the camera but didn't move? Did it switch to the camera view at all? Let me know.
Quote:
Oneofthe8devilz: here is an example of what you can do with the tool
Wow devilz that was awesome. The detail in the animation was unbelievable. There is no way a cinematic made in the editor can match what is possible in an md5anim. The possibilities are endless. I have a 3dsmax book, but it's the size of a phone book so I don't think I'll ever get around to learning it. I'll leave the md5anim's up to the pro's like you.

Quote:
Docgalaad: OMG I LOST 6 HOURS TO DEBUG A FUNCTION
I am so sorry Docgalaad! I should have worded it more clearly. Thank you for posting your solution. I hope you're not too mad at me.

indianajonesilm@Posted: Wed Sep 22, 2004 2:40 am : Updated on October 3, 2004
Creating a simple cinematic with a moving camera.
Hello, this is my first tutorial. I'm a newbie, so if you find any mistakes please let me know.
Step 1.
Lets start by making a small room and add an "info_player_start" and a light.
Step 2.
Add a "func_cameraview".
Step 3.
Next make a NURB spline path for the camera to follow and name it "camera_spline".
Read Rayne's excellent NURBS tutorial here:
http://www.doom3world.org/phpbb2/viewtopic.php?t=5123
Instead of moving a light around the room, we will be moving the camera along this path.
Step 4.
Create a "func_mover" and name it "anchor". You must add a Key of cinematic" with a Value of "1". This is very important if you want the camera to move. I also add the Key "solid" with the Value of "0" so the camera can pass through stuff. You should also texture this func_mover with the "common\nodraw" texture.
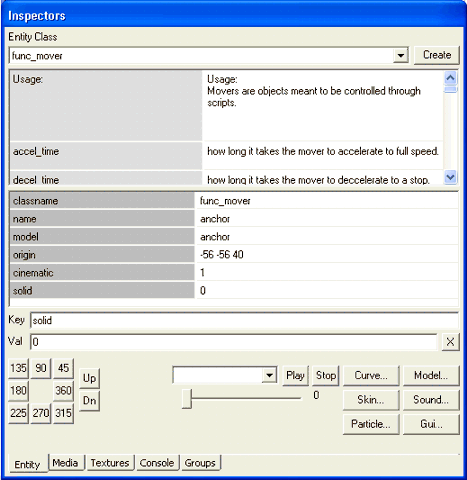
Step 5.
Next we need to bind the func_camera view to the func_mover. Select the "func_cameraview" and add the Key "bind" with a Value of "anchor" in the entity inspectors window.
Step 6.
Add a "trigger_once" and give it a Key "call" with a Value of "camera_script". This is the trigger that will call the script we make in the next step.
Step 7.
That's it, now we need to write a script to switch the view to the func_cameraview and start it moving along the spline. Open up notepad and type the following:
Code:
void start_camera_spline()
{
$anchor.time(10); //How many seconds it will take to follow the spline from start to finish
$anchor.accelTime(1); //How long it takes to get up to speed
$anchor.decelTime(1); //How long it takes to decelerate
$anchor.startSpline($camera_spline); //Start the func_mover "anchor" moving along the spline
}
void camera_script()
{
sys.setCamera($func_cameraview_1); //Set the view to the camera
start_camera_spline(); //calls the script above
sys.wait(10); //The time to wait before switching view back to normal
sys.firstPerson(); //Turn off the camera and switch back to normal view
}
Step 8.
Save the script above as "your map name.script" and save it in the same folder as your map. For example if your map is called "cave.map" then name your script "cave.script".
Step 9.
Compile your map and start it up! As soon as you touch the "trigger_once" the view will switch to the camera and follow the spline path. If you wanted to make an intro cinematic before your map starts then either place the trigger on your info_player_start or just connect(Ctrl-K) your info_player_start with a "trigger_relay" that calls the script.
Step 10 (and other cool stuff).
Remember to add the
Key "cinematic" with the Value of "1" to all your func_mover's, func_rotator's, characters, and other entities that you want to see move in your cinematic.
Be sure to add the key to all lights that are triggered in your cinematic also. Any entity that is used during the cinematic, has to have this key set. (thanks Docgalaad). You can add special camera tricks to your cinematic by playing with the FOV (frame of view) to get cool warp effects. You can have many different cameras and just switch the view to another one whenever you want by triggering that camera with script or a trigger. If this tut has helped you out and you want to thank me, just mention me in the end credits of your map!


Here is an example of a simple cinematic which I made using the editor.

Download the pk4 here:
http://webpages.charter.net/aldanafx/stuff/cinematic_example.zipPlace the "cinematic.pk4" in your base folder and type "map cinematic" in the console to start it.
Update October 3, 2004How to aim the moving camera.(Thanks to rich_is_bored and MBolus for the help with the script)
Step 1.
First we need something for the camera to aim at, so we will add a "target_null" to the map. Place the target_null anywhere you want, and the camera will continuously aim at it while it travels along the spline. The target_null doesn't have to be in a fixed position, it can be binded to a mover and move along it's own spline if you wish.
Step 2.
Next we need to add a "target_setkeyval" to the map. A target_setkeyval is used to set or change Key's and Values of a targeted entity. In this case we will be using this target_setkeyval to add a Key\Val pair to the func_camera view. Now connect (Ctrl-K) the "target_setkeyval" to the "func_cameraview".
Step 3.
Select the "target_setkeyval" and give it a Key of "keyval" and a value of "target;target_null_1". It should look likethe following picture:

Step 4.
Now we need to add some additional script to tell the camera to continually aim at the target_null while it moves along the spline. Here is the previous script from above with the new aiming script added in:
Code:
void start_camera_spline()
{
$anchor.time(20);
$anchor.accelTime(1);
$anchor.decelTime(1);
$anchor.disableSplineAngles(); //You need to add this when aiming a moving camera.
$anchor.startSpline($camera_spline);
}
//This is the new aiming script:
void update_camera()
{
sys.threadname("aim_loop"); // Gives this thread the name "aim_loop" so it can be killed later.
while (1)
{
//This triggers the target_setkeyvall which in turn adds a key/val pair to the func_cameraview:
sys.trigger($target_setkeyval_1);
sys.waitFrame(); //This waits for the next frame before continuing
}
}
void camera_script()
{
sys.setCamera($func_cameraview_1);
start_camera_spline();
thread update_camera(); // This calls the function update_camera as a thread so it
// runs in the background.
sys.waitFor($anchor); // This halts execution until anchor finishes it's movement.
sys.killthread("aim_loop"); // This kills the thread "aim_loop"
sys.firstPerson(); //Turn off the camera and switch back to normal
}
Step 5.
As you see above, it is nessecary to use the "disableSplineAngles" command in your script when tracking something with the moving camera.
That's it, your camera should now aim at the target_null while it moves along the spline.
rich_is_bored@Posted: Wed Sep 22, 2004 3:06 am : Ah!
* slaps forehead *
I tried this exact thing before. The only difference was i didn't know about this cinematic key/value pair.
Thanks alot!

I'll try this out soon and verify if you have any corrections to make.
MBolus@Posted: Wed Sep 22, 2004 3:43 am : I have also been using this type of thing, including around areas such as hallways with teleporters, and found it to really enhance a level. Fov of 140 gives a pretty wide indoors view, compared with 90.
I would add a few minor points. There are a few additional useful keys. For example, you can specify whether an entity will hide after its anims, or whether it will stay to potentially attack, and there is a key you could use for the func_cameraview to indicate whether non-cinematic entities with enemies will be seen. Also, if you preferred you could use triggers to start and stop the new camera view.
rich_is_bored@Posted: Thu Sep 23, 2004 4:49 am : I've just tested it out and it works great.

Now the only thing left is to figure out a way to aim the camera at a target while it moves on the spline.
Great work though.

abigserve@Posted: Thu Sep 23, 2004 5:53 am : let us know how that turns out rich

Rayne@Posted: Thu Sep 23, 2004 7:32 am : Oh bè, you have to connect the func_cameraview's to a target_null (when you connect them, the cam view in the editor switch from the cameraview entity).. so you have only to bind and move even the target_null

rich_is_bored@Posted: Thu Sep 23, 2004 8:11 pm : Your right, targeting the camera_view to a target_null aims the camera.
The problem is that while the camera is initially aimed at the target_null when the map loads, it doesn't follow the null should the camera or null's position change.
indianajonesilm@Posted: Thu Sep 23, 2004 8:57 pm : Quote:
Now the only thing left is to figure out a way to aim the camera at a target while it moves on the spline.
I'm working on it.

I hope there is a way to do it. I added a little example of a cinematic for everyone. (you're in the credits

) I hope my free isp webspace doesn't exceed it's bandwidth limit.
rich_is_bored@Posted: Thu Sep 23, 2004 9:40 pm : That was impressive.

I hope you have the bandwidth to handle all the traffic you're going to get.
elroacho@Posted: Fri Sep 24, 2004 1:41 am : works like a charm...
a question tho, i want to pause the camera at the end of the spline for a few seconds while a big platform(func_door) moves down. i trigger the platform and the speakers for the sound of the moving plat a few seconds into the cinimatic. i can hear the souds of the plat but the func_door dosen't move until the cinimatic has completed and i can move again.
is their something special i have to do to trigger it to play in a cinimatic? if i trigger it with out the camera the func_door and the sound start at the same time like it's supposed to.
1 more thing, lets say you have a trigger to the cinimatic right before a door. then you put in a "recycling2_mancintro_player" to show the charector walking in the cinimatic. how to you make it so that when the cinimatic finishes, the player starts at a different spot then where they triggered it? like the player triggeres cinimatic from the beginning postition of "recycling2_mancintro_player" and when it finishes, i want the player to be at the end postition of "recycling2_mancintro_player".
help please, i'm tring real hard

indianajonesilm@Posted: Fri Sep 24, 2004 2:11 am : Quote:
is their something special i have to do to trigger it to play in a cinimatic?
You have to give the door a Key of "cinematic" with a Value of "1" in the entities inspector window in oder to see it move in your cinematic.
Quote:
how to you make it so that when the cinimatic finishes, the player starts at a different spot then where they triggered it?
Try using a "info_player_teleport". Make a Trigger in the start location. Ctrl-K the trigger with the info_player_teleport. When the player touches the tigger, (you can give it the Key "visualView" with the Value "Camera Name") it will switch views to that camera for your cinematic. (Check the usage of the different Key's in the entities inspector window). After the cinematic is over, your player will be in the location of the info_player_teleport.
That might work.
elroacho@Posted: Fri Sep 24, 2004 7:16 am : awesome, worked perfectly
thanx indianajonesilm
abigserve@Posted: Fri Sep 24, 2004 8:33 am : 
not working 4 me....i followed the tut exactly as well...
Oneofthe8devilz@Posted: Fri Sep 24, 2004 9:18 am : this stuff is amazing !!!!
I think this method combined with the animation export tool from "der_ton" are the ideal fundament for making professional machinima movies.
here is an example of what you can do with the tool
http://www.doom3world.org/phpbb2/viewtopic.php?t=5539
So, for the difficult parts like going up the stairs one could remove the stiffness of the models by creating an md5anim.......
Docgalaad@Posted: Sat Sep 25, 2004 2:47 am : OMG I LOST 6 HOURS TO DEBUG A FUNCTION
You have to be
EXTREMLY CAREFUL about the
cinematic key when you wish to move objects in front of a camera !
I didnt know this hidden key/value pair. And to learn how to use a camera, i looked into one used in the game. Unfortunatly, this one didnt make objects to move in the scenery.
I did a function moving one object, and activating a light to have a better view of what's happening. Once the cinematic switch back to first person view, i set the light off again.
My script was perfect until i test it. The script entered in a weird loop, and the camera made the script to never terminate. The game was stuck in the cinematic. I scanned every line and did a ton of modification. Until i look into THIS THREAD on the forum. Worse, i knew this post, i already read it 3 days ago. But i completly passed through the cinematic key issue.
So, if you have this trouble, yes you, i expect you will search for those keyword on the forum:
CAMERA FUNC_MOVER CINEMATIC LIGHT
Why light ? Cause the light on in my cinematic was a part of it, somehow, and i had to add the key/value cinematic/1 on the light, to get out of my cinematic and make the whole script running properly.
Any entity that is used during the cinematic, has to have this key set.
Not only the moving objects. I hope it's clear cause i'm a bit upset about the lost time


Cheers.
wheelbarrow@Posted: Sat Sep 25, 2004 3:02 am : I have a cinematic with 8 lights, 2 of them flashing, and 7 func_emitters, and they all work fine without the 'cinematic' key/value. The only thing with this key is an opening door....
Docgalaad@Posted: Sat Sep 25, 2004 3:04 am : I trust you. But removing the key on the light and my cinematic is stuck forever. The map works perfectly with the key set.
Do you trigger your lights and emitters during the cinematic ?
Cause i do on my part. Maybe...
xflame@Posted: Sun Sep 26, 2004 11:50 pm : I had a strange problem with this, when I ran my file, I got the error:
CANNOT BIND TO ITSELF!
wheelbarrow@Posted: Mon Sep 27, 2004 12:45 am : No, I don't trigger anything but the door during the cinematic. As for the error message above, just like it says, you've tried to bind something to itself. I did this myself by accident a while ago. Just go thru your entities, and make sure you're not binding the mover to itself. Easy mistake, easy to fix

indianajonesilm@Posted: Mon Sep 27, 2004 1:46 am : Quote:
abigserve: not working 4 me....i followed the tut exactly as well...
Sorry abigserve, I'm new to writing tutorials and I'm still figuring out this editor stuff. I've only been using it for a few weeks. I apologize for my inability to write a decent tutorial. Maybe I can help figure out the problem you're having if you give me a little more info. Did the view switch to the camera but didn't move? Did it switch to the camera view at all? Let me know.
Quote:
Oneofthe8devilz: here is an example of what you can do with the tool
Wow devilz that was awesome. The detail in the animation was unbelievable. There is no way a cinematic made in the editor can match what is possible in an md5anim. The possibilities are endless. I have a 3dsmax book, but it's the size of a phone book so I don't think I'll ever get around to learning it. I'll leave the md5anim's up to the pro's like you.

Quote:
Docgalaad: OMG I LOST 6 HOURS TO DEBUG A FUNCTION
I am so sorry Docgalaad! I should have worded it more clearly. Thank you for posting your solution. I hope you're not too mad at me.

Docgalaad@Posted: Mon Sep 27, 2004 2:42 pm : Absolutly not your fault. I'm a noob in learning mode.
As i said, i copied a cameraview from the vanilla game.
Unfortunatly, there was no object moving in the scenery.
So ID didnt use the cinematic key for this camera. No luck.
Anyway, there is still something wrong in my setup.
Everyone seems to confirm there is no need for the key in light entities.
But in my cinematic, if the light does not get the key, the cinematic is screwed.
My map is working 100%. So no worries. But if really lights dont need the key, then there is something wrong in my map, even working as is though.
indianajonesilm@Posted: Tue Sep 28, 2004 4:12 pm : Quote:
Now the only thing left is to figure out a way to aim the camera at a target while it moves on the spline.
Well, I'm still working on it. I figured out how to aim the camera at any target null while it's moving on the spline by using a "target_setkeyval". You have to connect (Ctrl-K) the "target_setkeyval" to the "func_cameraview". Give the "target_setkeyval" a Key of "keyval" and a Value of"target;target_null_1" which will add the key "target" and the value of any "target_null" you want to switch views to. Then just trigger the target_setkeyval in your script when you want the camera view to change to the targeted null. You can change targets as much as you want during the camera movement on the spline, the only problem is that it will not stay fixed on the target_null.
Another neat thing you can do is rotate the camera in any direction while it's moving on the spline. You can even do spins, loops and rolls. For example if you wanted to rotate the camera 45 degrees to the right you could add this script:
Code:
void rotate_camera_right()
{
$anchor.time(3);
$anchor.accelTime(1);
$anchor.decelTime(1);
$anchor.rotateOnce('0 45 0');
sys.waitFor($anchor);
}
Then in the the main camera script just call this script above when ever you want it to rotate while it's moving on the spline.
Code:
rotate_camera_right();
I'll keep working on trying to figure out how to keep the aim fixed on the target null.
MBolus@Posted: Tue Sep 28, 2004 10:18 pm : As I mentioned previously, I have also been working with cameras in my experiments with monsters, including a nice zooming teleport cam, and the monster cam! Although I have described them briefly, I finally had the time to describe the latter in detail. I haven't heard of anyone else discussing anything like this, but I figured it might help you a bit, including considering how the monsters can aim the cameras. Hope this helps with your work too, as well as with the machinima projects, as this could be used by hooking to other entities as well!
http://www.doom3world.org/phpbb2/viewtopic.php?t=6356
indianajonesilm@Posted: Fri Oct 01, 2004 2:29 am : I figured it out!!! woohoo!!!! well, sorta. I figured out how to keep the camera aimed at a target (even if the target is moving) while it travels on the spline, but I just need some help with the script.
I made a loop so that the camera constantly snaps it's view to the target_null and it does it so fast that it's really smooth and my fps stays at a steady 60 fps. The problem is that I suck at scripting and I don't know how to stop the loop once the mover(anchor) reaches the end of the spline.
I used a "target_setkeyval" to set the key/val pair of the camera to target the "target_null" and I just trigger it in script with a loop:
Code:
void start_camera_spline()
{
$anchor.time(20); //How many seconds it will take to follow the spline from start to finish
$anchor.accelTime(1); //How long it takes to get up to speed
$anchor.decelTime(1); //How long it takes to decellerate
$anchor.startSpline($camera_spline); //Start the func_mover "anchor" moving along the spline
}
void camera_script()
{
sys.setCamera($func_cameraview_1); //Set the view to the camera
start_camera_spline(); //calls the script above
//Need to figure out how stop the loop once the $anchor reaches the end of the $camera_spline
while (1)
{
sys.trigger($target_setkeyval_1); //This tells the camera to point to the target_null
sys.wait (.01);
}
sys.firstPerson(); //Turn off the camera and switch back to normal
}
If someone could help me out with this script I would be most grateful. Thank you.
rich_is_bored@Posted: Fri Oct 01, 2004 5:05 am : You could place that loop in it's own function. Then you could thread the function so the camera updates in the background and kills the thread when the cinematic is over.
Here's some rough modification of the code you posted...
Code:
void start_camera_spline()
{
$anchor.time(20);
$anchor.accelTime(1);
$anchor.decelTime(1);
$anchor.startSpline($camera_spline);
}
void update_camera()
{
sys.threadname("foo"); // Gives this thread the name "foo" so it can be killed later.
while (1)
{
sys.trigger($target_setkeyval_1);
waitFrame(); // Changed this so it's not time dependant but rather frame dependant.
// This should make the snapping as fluid as possible.
}
}
void camera_script()
{
sys.setCamera($func_cameraview_1);
start_camera_spline();
thread update_camera(); // This calls the function update_camera as a thread so it
// runs in the background.
sys.waitfor($anchor); // This halts execution until anchor finishes it's movement.
killthread("foo"); // This kills the thread "foo", which by this time, should be
// running the update_camera loop.
sys.firstPerson(); //Turn off the camera and switch back to normal
}
I'd test this myself but it's getting kinda late and I'll be off to bed soon.
If you don't get to it before me I'll try this out in the morning.
MBolus@Posted: Fri Oct 01, 2004 6:05 am : I agree with Rich, except for minor things such as syntax: you should change waitFrame to sys.waitFrame and killthread to sys.killthread , because these are system events. Also, you should change waitfor to waitFor , because it the system can be persnickety.
This will work well with these minor changes. You probably would have figured this out anyway, but I happened to notice this post and wanted to be helpful.
rich_is_bored@Posted: Fri Oct 01, 2004 6:12 am : Like I said, it was a rough edit. I hadn't tested it.

MBolus@Posted: Fri Oct 01, 2004 6:13 am : Understood.

indianajonesilm@Posted: Fri Oct 01, 2004 6:54 am : YOU GUYS ROCK!!!!! Thank you all for your help, you have no idea how much I appreciate everyone taking time to help out others like myself. What a great place this is. I've had so much fun learning new things from helpful people like yourselves. Thank you again, you guys are great.
One more thing to add. If you find that the camera starts going crazy around corners or going up and down the spline, just add "disableSplineAngles" like this:
Code:
$anchor.time(20);
$anchor.accelTime(1);
$anchor.decelTime(1);
$anchor.disableSplineAngles(); //add this if you get crazy camera angles
$anchor.startSpline($camera_spline);
Docgalaad@Posted: Fri Oct 01, 2004 1:19 pm : I saw some screenies of your work indy, and i'm glad some peoples helped you out with the camera problem. This is promising.
I'm also working with a subversion of this code example, damn you rocks, my head is full of ideas now.
About the crazy angles, i found i have better results if the spline uses only 2 axis at a time during its evolution. I mean, at every point, one axis position should remain the same.
rich_is_bored@Posted: Fri Oct 01, 2004 6:14 pm : From what experimenting I've done it seems like using that disableSplines command is nessecary if you plan to track something with the camera, let alone stopping crazy movement.
Anyway, glad to see we got this worked out.

xflame@Posted: Fri Oct 01, 2004 11:38 pm : Okay, I got the everything working, no errors or anything, but for my test cinematic, my camera wont even start! Can someone help a poor Doom newbie as myself?

What happens is that when I start my map, I just start out as a person, without the camera starting!!!
One more question: I feel dumb and stupid asking this, but how add a trigger_once?
indianajonesilm@Posted: Sat Oct 02, 2004 8:31 pm : Quote:
One more question: I feel dumb and stupid asking this, but how add a trigger_once?
You add a trigger_once by right clicking on the screen (the grid part) and select trigger\trigger_once. Then give it a Key of "Call" with the Val of "camera_script" in the entity inspectors window. If you don't have a trigger the view will not switch to the camera.
bad marine ass@Posted: Sun Oct 03, 2004 5:16 am : It doesn't work for me damn. I don't intend to have a moving camera, so I only added the second half of the camera script into the .script file. I also just added a trigger_once and gave it a key "call" with value "camera_script". Am I doing anything wrong here? I can't go into the camera's view
BladeBezerker@Posted: Sun Oct 03, 2004 5:26 am : I cant get speakers to work. Ive got the camera and everything, but I cant get sound to play unless I use start sound shaders. These are fine, but they stop after the cinematic ends. I was the sound to keep playing when it ends. How do I do this?
TodT7@Posted: Sun Oct 03, 2004 10:38 am : indianajonesilm wrote:
You add a trigger_once by right clicking on the screen (the grid part) and select trigger\trigger_once. Then give it a Key of "Call" with the Val of "camera_script" in the entity inspectors window. If you don't have a trigger the view will not switch to the camera.
I've figured out that
trigger_once which starts the cinematic
must have the key "
target" pointing to (according to this tutorial)"
func_cameraview_1".
If trigger don't have such a key, view dosn't switch to the camera.
indianajonesilm@Posted: Sun Oct 03, 2004 9:11 pm : Quote:
bad marine ass: It doesn't work for me damn. I don't intend to have a moving camera, so I only added the second half of the camera script into the .script file. I also just added a trigger_once and gave it a key "call" with value "camera_script". Am I doing anything wrong here? I can't go into the camera's view
Make sure that the camera name in the script matches the name of the camera you want to switch views to. If you pull down the console, sometimes it tells you what the error is. A lot of times it's just something spelled wrong or a lower case letter instead of an upper case. Also try making a bigger tirgger(you need to touch the trigger for it to work) or use a trigger relay to call the script.
Code:
void camera_script()
{
sys.setCamera($func_cameraview_1); //set this to the name of the func_cameraview in your map
sys.wait(10); //The time to wait before switching view back to normal
sys.firstPerson(); //Turn off the camera and switch back to normal view
}
Quote:
BladeBezerker: I cant get speakers to work. Ive got the camera and everything, but I cant get sound to play unless I use start sound shaders. These are fine, but they stop after the cinematic ends. I was the sound to keep playing when it ends. How do I do this?
I made a test map and tested the speakers. All the speakers worked fine. I also tested a speaker with a looping sound that was triggered in the cinematic and it also worked fine and contiued to work fine after the cinematic was over. I also testing a speaker that started before the cinematic and it continued to work during and after the cinematic. I don't know why you're having problems, sorry. Maybe someone else might have a solution for you.
Quote:
TodT7: I've figured out that trigger_once which starts the cinematic must have the key "target" pointing to (according to this tutorial)"func_cameraview_1".
If trigger don't have such a key, view dosn't switch to the camera.
Well actually you don't need to have the trigger target the camera. You just need it to call the script. The script switches the view to the camera with this line:
Code:
sys.setCamera($func_cameraview_1); //Set the view to the camera
You could use a trigger if you wanted but if you have multiple cameras it's easier to switch between them by using script.
I think it would be very useful if everyone who is having problems would download the example cinematic that I made and open it up in the editor and see how I did things. I think that would answer a lot of your questions. I hope this helps.
BladeBezerker@Posted: Sun Oct 03, 2004 10:50 pm : Maybe I'm using the wrong code for triggering the speaker. Im a total noob at scripting (NEVER done it before, I dont know any programming languages or anything). Also the example map didnt use any speakers.
Can someone tell me the code for triggering the speaker. This is my whole maps code at the moment:
Code:
void start_camera_spline()
{
$anchor.time(15); //How many seconds it will take to follow the spline from start to finish
$anchor.accelTime(1); //How long it takes to get up to speed
$anchor.decelTime(10); //How long it takes to decelerate
$anchor.startSpline($camera_spline); //Start the func_mover "anchor" moving along the spline
}
void camera_script()
{
sys.setCamera($func_cameraview); //Set the view to the camera
start_camera_spline(); //calls the script above
sys.trigger( $scape5 ) ;
sys.wait(18); //The time to wait before switching view back to normal
$Eyeslight.On();
$Eyes1.On();
$Eyes2.On();
sys.wait(2);
sys.firstPerson(); //Turn off the camera and switch back to normal view
}
Eyeslight is my light, Eyes1 and 2 are partcile effects that I cant get working, so if anyone knows why please help, and scape5 is my speaker. The speaker has cinematic, its at 100 volume, but i still cant hear anything. So if anyone can tell me the right code for it thanx alot.
indianajonesilm@Posted: Mon Oct 04, 2004 1:49 am : I've updated the tutorial with "How to aim a moving camera".
BladeBezerker, try using the Sound Editor. Goliath has a great video tutorial on how to use it. Look for it in the Tutorial list
http://www.doom3world.org/phpbb2/viewtopic.php?t=3017
jgebbeken@Posted: Tue Oct 05, 2004 11:20 pm : I am not sure what I am missing something but does anyone have a pk file of there map so I can see why I can't get the cam to point at what I need it to point at?
xflame@Posted: Tue Oct 05, 2004 11:22 pm : I looked at your map, and sae you had to target a target_null, why did you do this? just wondering
Also, when my camera runs, there is a black square in the middle that blocks some of the view, how do you eliminite that.
indianajonesilm@Posted: Wed Oct 06, 2004 12:14 am : Quote:
jgebbeken: I am not sure what I am missing something but does anyone have a pk file of there map so I can see why I can't get the cam to point at what I need it to point at?
I made a quick test map for you so you can see how I aimed the moving camera at a moving target. The "target_null" is binded to the mover bird.

Download the PK4 here:
http://webpages.charter.net/aldanafx/stuff/aim_example.zipQuote:
xflame: I looked at your map, and sae you had to target a target_null, why did you do this? just wondering
Also, when my camera runs, there is a black square in the middle that blocks some of the view, how do you eliminite that.
I have a target_null in that map because I used it to aim a camera that is not moving. If you have a black square in your map, make sure you texture your func_mover "anchor" and your func_static "camera_spline" with the "nodraw" texture. I hope this helps.
jgebbeken@Posted: Wed Oct 06, 2004 1:43 am : I think I found the problem. I didn't put my $anchor.disableSplineAngles(); before the $anchor.startSpline($spline1); Plus I didn't call the thread aim_loop so threw the whole thing off.
now how do you get that same camera to travel on another mover?
thanks for your help

indianajonesilm@Posted: Wed Oct 06, 2004 4:38 am : jgebbeken wrote:
now how do you get that same camera to travel on another mover?
There are many different ways to achieve that depending on the situation. You could trigger a "target_setkeyval" to change the camera's bind value to a different mover or you could just make the same mover travel along a different spline. You could also just make a different camera and switch camera views.
bad marine ass@Posted: Thu Oct 07, 2004 1:28 pm : ok heres a more advanced question for my own intelligence lol. i cant figure out how to make the cameras fade in and out
ok see, what im trying to do is to create 3 rooms with different "worded" textures on them. i have placed 3 cameras into these 3 rooms, and connected each of them to a trigger_fade respectively. i can make the camera target only the first room; after that, i have no idea how to make the camera fade out and then go into the eyes of the second camera to view the second room, and finally to go into the eyes of the third camera to view the third room
can anyone help me please?
BladeBezerker@Posted: Thu Oct 07, 2004 10:42 pm : Ok I still cant get my speakers to work so Ive uploaded my map, I changed the textures so I wouldn't have to upload 10 megs of them, so the map doesnt really look this bad. Download the map at
http://members.optusnet.com.au/~nicwix/Gothic.pk4
If you can fix anything please post how you did it. Thanks!
indianajonesilm@Posted: Fri Oct 08, 2004 12:51 am : Okay, time for some more Q&A

Quote:
bad marine ass:i have no idea how to make the camera fade out and then go into the eyes of the second camera to view the second room, and finally to go into the eyes of the third camera to view the third room
Use script to trigger the fade with something like:
Code:
sys.trigger($name of your trigger_fade here);
and then trigger a different cameraview with:
Code:
sys.setCamera($name of camera here);
Quote:
BladeBezerker:: Ok I still cant get my speakers to work so Ive uploaded my map, I changed the textures so I wouldn't have to upload 10 megs of them, so the map doesnt really look this bad. Download the map at
http://members.optusnet.com.au/~nicwix/Gothic.pk4If you can fix anything please post how you did it. Thanks!
Ok, this is not really a speaker tutorial, but I'll help you out the best I can. I downloaded your map. Damn, you had a lot of things wrong with your map!!!

Where should I start.
1. If you want to trigger a speaker, you have to have the Key "s_waitfortrigger" and a Val or "1", you did not have this.
2. You had a trigger_once triggering the speaker in your map, and you also were triggering the speaker in the script again which would turn it off right away. Just trigger it in the script.
3. You had TWO trigger_once's on top of each other on top of the info_player_start. what I mean is they were all in the same location. huh??? why?
4. You had a speaker with no soundshader selected for it, so I just got rid of it.
5. I got rid of all those "Trigger_once"'s that you had and just put a single "trigger_relay" there beside the info_player_start. I selected the info_player_start and then selected the trigger_relay and then hit Ctrl-K. Now select the trigger_relay and give it a Key of "call" and a Val of "camera_script". that's it.
You now have a working speaker. I hope this helps.

BladeBezerker@Posted: Fri Oct 08, 2004 2:49 am : Thanks, this is the first ever map Ive made for anything like this. I love the editors, if I knew about FPS editors I would have done it much sooner. Thanks, put 2 trigger_onces, because I tried with one and it didnt work (this was another trigger) and it worked when I made 1 trigger_once for each action.
Could you also have a look at why my particles arent turning on? I've tried everything , I can trigger them in game but not via script - help!
bad marine ass@Posted: Fri Oct 08, 2004 7:26 am : w00t thanks indianajonesilm!
bad marine ass@Posted: Fri Oct 08, 2004 9:22 am : ok still doesnt work quite right for me. Basically the camera fades in to room #1, then it fades out (total blackness) and doesnt move on to room 2 and 3. Here's the code
Code:
void intro()
{
sys.trigger($trigger_fade_1); //Triggers the fading
sys.setCamera($func_cameraview_1); //Set the view to the camera
sys.wait(10);
sys.trigger($trigger_fade_3);
sys.setCamera($func_cameraview_3);
sys.wait(10);
sys.trigger($trigger_fade_4);
sys.setCamera($func_cameraview_4);
sys.firstPerson();
}
indianajonesilm@Posted: Fri Oct 08, 2004 10:37 am : Quote:
BladeBezerker: Could you also have a look at why my particles arent turning on? I've tried everything , I can trigger them in game but not via script - help!
I see that you started another thread about your trigger/particles problem. If no one has helped you out by tomorrow, I'll take a look at it for you and post in the other thread.
I want to keep this thread about cinematics. Quote:
bad marine ass: ok still doesnt work quite right for me. Basically the camera fades in to room #1, then it fades out (total blackness) and doesnt move on to room 2 and 3.
Ok since fading in and out of camera views does pertain to cinematics then I'll help you out. I see in your script that you faded out, but you didn't fade back in. Here is exactly what you need to do:
1. You only need two trigger_fades, one to fade in and another to fade out. You don't need the cameras to target them. So, add a trigger_fade and give it the name "fade_in". Give this trigger_fade a fade color of "0 0 0 0" and a fade time of "3"
2. Now add another trigger_fade and give it a name of "fade_out" with a fade color of "0 0 0 1" and a fade time of "3" seconds.
3. Use this script that I modified from your own script:
Code:
void intro()
{
sys.trigger($fade_in); //trigger_fade with fade color 0 0 0 0
sys.setCamera($func_cameraview_1); //Set the view to the camera
sys.wait(10); //how long to stay on this camera
sys.trigger($fade_out); //fade color 0 0 0 1
sys.wait(3); //how long the fade takes (fadeTime val)
sys.setCamera($func_cameraview_2);
sys.trigger($fade_in); //tirgger_fade with fade color 0 0 0 0
sys.wait(10); //how long to stay on this camera
sys.trigger($fade_out); //trigger_fade with fade color 0 0 0 1
sys.wait(3); //how long the fade takes (fadeTime val)
sys.setCamera($func_cameraview_3);
sys.trigger($fade_in); //tirgger_fade with fade color 0 0 0 0
sys.wait(10); //how long to stay on this camera
sys.trigger($fade_out); //trigger_fade with fade color 0 0 0 1
sys.wait(3); //how long the fade takes (fadeTime val)
sys.trigger($fade_in); //tirgger_fade with fade color 0 0 0 0
sys.firstPerson();
}
4. Make sure the camera names are the camera's you want to switch to. That's it. It should fade in and out of all your camera views.
I'm going to get some sleep. I hope this helps.

bad marine ass@Posted: Fri Oct 08, 2004 11:10 am : You, sir, are a genius
I wish I could repay you in kind, but oh well...would an online kiss suffice?

Thanks, I've finally done my cinematics!

heXum@Posted: Fri Oct 08, 2004 6:12 pm : I didn't read the whole thread so I don't know if someone said this already... but everything here can be done via entities in the editor and key/values. No scripting involved. Look at the options per entity in the editor, there's a ton of things you can do.
BladeBezerker@Posted: Sat Oct 09, 2004 1:42 am : its strange, once I got the speakers to work, the particles worked too, I have no idea why they werent working or are suddenly working but they are. Don't worry.
Wolf@Posted: Tue Oct 12, 2004 5:28 pm : Greetings Indianajoneslim!
I have been lurking and learning, I admit it, with no shame whatsoever

. Many thanks for your tutorial which has allowed me to do some very nice camera moves. I am at a loss on how to control camera "rolls" following a spline though and need your help in finding a solution.
I can control a camera roll (how much roll and for how long) very well, as long as the spline is straight. As soon as I modify the path of the straight spline that affects the z axis (any curves whatsoever), I end up with crazy camera movement. I cannot use "disableSplineAngles" which solves the crazy movements (as specified earlier in this thread) cause I need the camera to follow the spline as is. I have not found any solution to this, after many sleepless days and nights. Please... help!!!
Conclusion: I want to be able to roll the camera in the direction of the curve of the spline like a racing car going around the track. Does this make any sense??
indianajonesilm@Posted: Wed Oct 13, 2004 2:07 am : Quote:
bad marine ass: Thanks, I've finally done my cinematics!
Great! You're welcome.
Quote:
heXum: everything here can be done via entities in the editor and key/values. No scripting involved.
A lot of it can be done without script but I don't thing you can aim a moving camera at a moving target without script. If you know how, please share it with us.
Quote:
BladeBezerker: ts strange, once I got the speakers to work, the particles worked too, I have no idea why they werent working or are suddenly working but they are. Don't worry.
Yeah, it was just a problem with your triggers, the script you wrote was fine. Once we got the script called, everything worked fine. I like your map, nice stain glass windows.
Quote:
Wolf: I am at a loss on how to control camera "rolls" following a spline though and need your help in finding a solution.
Ok so you want to roll a camera while it travels along the spline, sort of like a rollercoaster doing a barrel roll on it's tracks while the tracks are curving? If that's what you mean then you need to add a "func_rotating" to your map. Bind your cameraview to the func_rotating and then bind the func_rotating to the func_mover "anchor". Here is an example map for you to look at:
http://webpages.charter.net/aldanafx/stuff/roll_example.zip
I hope this helps.
Edit:
If you just want to bank the camera without rotating it all the way around, you don't need the func_rotater. Just put another mover in between the camera and anchor, and rotate this mover instead of anchor. Here is an example of banking the camera around a curve:
http://webpages.charter.net/aldanafx/stuff/bank_example.zip
doom3troubler101@Posted: Tue Nov 30, 2004 3:23 am : Ok here's my problem:
I have followed the tutorial as best as I could, i thought i had everything down pat but as soon as I start up my map and go into the console it shows me this warning message:
" trigger 'trigger_once_1' at (-218 0 -16) calls unknown function
'camera_script ' "
ANY help on this problem would be greatly appreciated, thanks in advance.
Enforcer@Posted: Tue Nov 30, 2004 4:17 am : make sure your trigger names match up to the script names, ive had problems like that before!
doom3troubler101@Posted: Wed Dec 01, 2004 3:32 am : One more question. When I put call with a value of camera_script, do I actually type camera_script or do I type camera_map.script or anything. For example my map name is tst.map, should I put camera_tst.map. I know this question may sound dumb, but i'm willing for any problems I may have made.
Oh and I checked my script and everything seems to be fine. One thing I noticed was that my func_cameraview didn't have a model, is it supposed to?
MBolus@Posted: Thu Dec 02, 2004 1:06 am : doom3troubler101 wrote:
but as soon as I start up my map and go into the console it shows me this warning message: " trigger 'trigger_once_1' at (-218 0 -16) calls unknown function 'camera_script ' "
First of all, you saved your script file as tst.script in the same directory as your tst.map, right?
doom3troubler101@Posted: Fri Dec 10, 2004 9:19 pm : Yeah, I did in the first place. I used windows customary "Notepad" and cut and copy'd the tutorial's script, as did I check my camera_spline name and everything so that it matched the code. The script is currently tst.script in the doom/base/maps... along with my tst.map, tst.proc, tst.cm, tst.bak, tst.aas96 and tst.aas48
Totally confused here... waiting for the right answer, but most people don't read to pg. 3 on this topic.
indianajonesilm@Posted: Mon Dec 13, 2004 7:49 pm : Ok, your trigger seems to be working because it does call the function camera_script but it doesn't find it. It's searching your tst.script file (which should be in the same folder as your tst.map file) for the following line:
Code:
void camera_script()
{
}
Then it does whatever is in between the "{ }". So if it's not finding the camera_script function then check the following:
1. In the editor, select the trigger you used to call the script and make sure the Key "Call" has a "Val" of "camera_script" and that none of the letters are capitalized so that it matches with the function name in your script.
2. Make sure you saved your tst.script file correctly. Make sure Windows is not hiding file extensions because you could be saving it as tst.script.txt without even knowing it. If the script file has a little blue notepad icon then it probably saved it as a .txt file.
3. Hmm, what else can I think of... Well, it's probably a good idea to download the cinematic example file and take a look at it and see how I did things in my map and see if you did them the same way. That might help.
I hope you get it working.
doom3troubler101@Posted: Fri Dec 17, 2004 6:22 am : indianajonesilm wrote:
2. Make sure you saved your tst.script file correctly. Make sure Windows is not hiding file extensions because you could be saving it as tst.script.txt without even knowing it. If the script file has a little blue notepad icon then it probably saved it as a .txt file.
Thank you sooo much, I can't beleive I missed this problem, It was annoying me big time, because without cinematics my maps were kinda dull. Once again thank you for pointing out this problem, and for your time.

Finko@Posted: Mon Aug 08, 2005 12:45 am : Ok, got it all working. Thanks a lot!!
One question...how do I make the player visible from the camera view. I placed a key 'cinematic' with value '1' on the player start, but no luck. Do i have to spawn a player model in or something? If so, I'll just find a tutorial to get that accomplished.
CraiZE@Posted: Wed Sep 07, 2005 8:36 pm : I would like to know why it happens that when i switch from cam1 to cam2, that i get an odd hick-up.
For example, you can look
here
(Map and Script Included) , and sorry for the linkage, but i didnt want to double post. But yet i thought i could find more help overhere

Hope i can finally get the bug sorted here. Oh and btw, it happens if both cams have the same target and aswell happens if they have different targets. But it only happens when switching the cams.
Actually, its like it isnt looking at the target, and then whoop quickly looks at it. Very annoying if you want to make a multiple timed camera path.
Ah well one of you might know! *crosses fingers* thanks in advance

chuckdood@Posted: Thu Sep 08, 2005 5:11 am : k, got it to work.
The problem was that your anchor2's origin was not the same as your spline origin. So, when the camera was switched, it is bound to anchor2's origin. As soon as you start moving anchor2 along Spline2, the origin of anchor2 is moved to the origin of the entity which contains the coordinates of spline2. You follow me? In the caes of your map, it was like 8, or 16 units above the origin of the spline.
So as soon as the switch occurred
a) the camera jumped from the end of spline1 up to Anchor2's origin
b) then Anchor2 (camera is bound to it) suddent jumps down to Spline2's origin
c) THEN starts moving along the spline.
all of this occurs in like a frame or 2.
ok. just check out the map file. I see no hickup with this, and it's using 2 cameras.
i included an updated script which contains code to print the movements to the screen.
http://home.comcast.net/~matkatamibakund/craize.zip
So, yeah. when you build your camera scenes:
1) put your mover at the same origin as your spline
2) don't make noticable changes in the angle of splineA's end, and splineB's start. it should look like a seemless transition from spline1 to spline2
Code:
void getViewAngle(string Ent);
void update_camera( string Ent)
{
sys.threadname("aim_loop");
while (1)
{
sys.trigger($target_setkeyval_1);
sys.waitFrame();
getViewAngle(Ent);
}
}
void start_camera_spline()
{
sys.print("using camera1's spline\n");
$anchor.time(8);
$anchor.accelTime(1);
$anchor.decelTime(1);
$anchor.disableSplineAngles();
$anchor.startSpline($camera_spline);
}
void start_camera_spline2()
{
sys.print("using camera2's spline\n");
$anchor2.time(8);
$anchor2.accelTime(1);
$anchor2.decelTime(1);
$anchor2.disableSplineAngles();
$anchor2.startSpline($camera_spline2);
}
void printInfo(string Ent, vector a, vector o )
{
sys.print("^3" + Ent + " Angles: " + a_x + ", " + a_y + ", " + a_z + "\n");
sys.print("^3" + Ent + " Origin: " + o_x + ", " + o_y + ", " + o_z + "\n");
}
void getViewAngle(string Ent)
{
/*
1. get the position of entA. (target_null_1)
2. get position of entB. (anchor)
3. calculate vector between them (entA_x - entB_x = Angle_x, etc..);
4. use Angle to print to screen.
*/
vector a, b, Angle;
entity ent;
ent = sys.getEntity(Ent);
a = $target_null_1.getWorldOrigin();
b = ent.getWorldOrigin();
Angle_x = a_x - b_x;
Angle_y = a_y - b_y;
Angle_z = a_z - b_z;
printInfo("Camera2 ", Angle, b);
}
void camera_script()
{
sys.setCamera($func_cameraview_1);
thread update_camera("anchor");
start_camera_spline();
sys.waitFor($anchor);
sys.wait(2);
sys.killthread("aim_loop"); //kill the thread using "anchor"
thread update_camera("anchor2"); //restart it using "anchor2"
sys.setCamera($func_cameraview_2);
start_camera_spline2();
sys.waitFor($anchor2);
sys.killthread("aim_loop");
}
CraiZE@Posted: Thu Sep 08, 2005 9:32 am : Ah yesh, you did it ! You're the champion *applause*
Thanks a bunch, now i can finally work on my cameras without hickups ;D
13579~@Posted: Fri Aug 03, 2007 2:47 am : Ok, sorry for posting in this ancient thread, but this is really pissing me off.
I made the test room, did everything exactly the way he showed, and it worked.
BUT, with or without the key/val pair cinematic/1 my guy won't show up in the camera view.
Also, monsters show if you don't add that key/val pair. So whats the point of adding it?
So, my question is, how do I get my player to appear?
Also wondering if this has anything to do with me not having 1.3.1 patch.
Even if it did, why does the monster show up without "cinematic" "1"?
This is really confusing me.
EDIT: Monsters only appear without cinematic 1 when they have team set to 0.
Also, if I make a monster who doesn't have cinematic 1 and also doesn't have team 0, if I run before I get attacked into the trigger once the camera will start without the monster. But when the camera is done, the monster doesn't reappear.
This is really confusing me.....why is this happening....
chuckdood@Posted: Fri Aug 03, 2007 3:00 am : wow, yeah, that is an ancient thread. Try updating to the latest version of the engine first, before you do anything else. I haven't played with doom3 for over a year, so my memory on how we got the camera thing to work is really hazy.
13579~@Posted: Fri Aug 03, 2007 3:17 am : chuckdood wrote:
wow, yeah, that is an ancient thread. Try updating to the latest version of the engine first, before you do anything else. I haven't played with doom3 for over a year, so my memory on how we got the camera thing to work is really hazy.
I'll try, but it'll take awhile.
I'm on dialup, so 1.3.1 is going to take about 4 hours.... downloading at 3.5kb/s
and it likes to disconnect fairly often...
Dogstar@Posted: Mon Dec 17, 2007 7:13 pm : Fantastic thread. One of my favourite in the collection of tutorials. Anyone wanting to make movies with Doom3 should really spend some time reading this lot and trying it out.
I got my scene to work perfectly (it IS really important to pay close attention to the tutorial and treble-check everything, though

).
I'd just like to know a couple of things:
1. Can I just have the map open straight into the moving camera view without a trigger_once (which I have to walk into) as per the tutorial? I'd like this to create purely cinematic scenes with everything camera-controlled (i.e. no need to physically move the play into the trigger).
2. What needs to be added to the script (
and where) to give the camera a FOV (field_of_view) of 60? This is normally "g_fov 60" in the console, but I'm guessing it's going to be different in the script.
This is the script I'm using:
Code:
void start_camera_spline()
{
$anchor.time(20);
$anchor.accelTime(1);
$anchor.decelTime(1);
$anchor.disableSplineAngles(); //You need to add this when aiming a moving camera.
$anchor.startSpline($camera_spline);
}
//This is the new aiming script:
void update_camera()
{
sys.threadname("aim_loop"); // Gives this thread the name "aim_loop" so it can be killed later.
while (1)
{
//This triggers the target_setkeyvall which in turn adds a key/val pair to the func_cameraview:
sys.trigger($target_setkeyval_1);
sys.waitFrame(); //This waits for the next frame before continuing
}
}
void camera_script()
{
sys.setCamera($func_cameraview_1);
start_camera_spline();
thread update_camera(); // This calls the function update_camera as a thread so it
// runs in the background.
sys.waitFor($anchor); // This halts execution until anchor finishes it's movement.
sys.killthread("aim_loop"); // This kills the thread "aim_loop"
sys.firstPerson(); //Turn off the camera and switch back to normal
}
Thank you!
chuckdood@Posted: Tue Dec 18, 2007 8:46 am : regarding #1, I would open up one of the ID levels that started with a cinematic, and see how they did it. Mars_City is that way. it's probably something you set in the Worldspawn entity, but don't quote me on that. I haven't played around in D3 for a few months.
KC Clark@Posted: Fri Feb 29, 2008 11:04 pm : I've been playing around with cinematics lately and would like to know how to add the player marine into the camera shot. I mean the way id did it by going into the back of his head and switching to first person. I posted this question in another thread and received an answer, however, it didn't really give the result I was looking for. And yes, I know this is an old post but I just got here.
chuckdood@Posted: Sat Mar 01, 2008 7:55 am : id Maps use custom animated player models that are triggered for the scene, and made to appear in the scene via the cinematic spawnflag.
I'd check out the EnPro map, as that has a good opening cinematic sequence that does what you are asking about.
Doomguy87@Posted: Sun Jun 22, 2008 6:52 pm : nvm
Doomguy87@Posted: Tue Sep 02, 2008 12:37 am : i dont know if this has been tried but is there a way to get videos into the game (non-cinematics) like .avi, .wmv files ect.
rich_is_bored@Posted: Tue Sep 02, 2008 3:16 am : You have to convert it to an ROQ. Then the game will be able to play it.
Look for a program called switchblade roq converter.
Brain Trepaning@Posted: Tue Sep 02, 2008 4:30 am : Download this file:
http://www.trepaning.com/roq.zip, extract to any folder.
create your DivX AVI at a power of 2 size, such as 256x512, or 512x512, and make it 30 frames per second.
Drag your AVI on to the program 1-sbCodebooks.exe.
When conversion is complete, drag the same AVI on to the program 2-sbvideo4.exe.
This is the .MTR you need to make to use the RoQ as a texture:
Code:
myRoQ
{
qer_editorimage textures/editor/video.tga
{
videoMap sound/roq/tunnelVision2001.roq
}
}
(I place my RoQ files in the SOUND folder for convenience, as the audio is a separate file from the video.)
Make a WAV of the audio and convert it to an OGG file with a free program like the audio editor AUDACITY. Place it in the same folder as the RoQ file. I am not certain, but if placed in the same folder, the audio may play automatically so long as it has the same name as the RoQ movie, but that may not be the case. Because I am not certain, write a SNDSHDR entry like:
Code:
myRoQ {
volume 0
global
sound/roq/tunnelVision2001.ogg
}
Finally, here is the code for a GUI overlay. I am no well-versed in their creation, so there may be more info than required here. Regardless, it works for triggering a movie and its audio. Note the COMMENTS in the code, as onTrigger and onAction do different things and can be swapped depending on what is wanted for an effect.
Code:
windowDef Desktop
{
rect 0 ,0 ,640 ,480
backcolor 0, 0, 0, 1
matcolor 0, 0, 0, 1
background "guis/assets/common/bg"
windowDef LocationText
{
rect 10,408,619,79
text "gui::gui_parm1"
textscale 0.94999999
textalign 1
forecolor 0.72941178,0,0,0
visible 1
background "guis/assets/main.tga"
matcolor 1,1,1,1
}
windowDef VidFrame
{
rect 0,0,640,480
visible 1
backcolor 1, 1, 1, 0
windowDef RoqFile
{
rect 0,0,640,480
visible 1
background "myRoQ" //video requiring RoQ mtr shader, place RoQ in sound/roq
matcolor 1,1,1,1
onTrigger //onAction clickable GUI activates individual GUI, onTrigger non-clickable triggered GUI activates all instances of GUI in map
{
resetCinematics ;
resetTime "Anim" "0" ;
localsound "tunnelVision2001"; //sound shader, place OGG in sound/roq, add to SNDSHD file
}
}
windowDef RoqFile1
{
rect 0,0,640,480
visible 1
background "guis/assets/launch"
matcolor 1,1,1,1
}
}
windowDef Anim
{
rect 0,0,0,0
notime 1
onTime 0
{
transition "RoqFile1::matcolor" "1 1 1 1" "1 1 1 0" "50" ;
}
onTime 24000 //1000 is 1 second
{
transition "RoqFile1::matcolor" "1 1 1 0" "1 1 1 1" "500" ;
}
}
}
Doomguy87@Posted: Tue Sep 02, 2008 11:41 pm : ok thanks guys, that sounds good also but i was hoping to make an intro video to play before the first map starts

Brain Trepaning@Posted: Wed Sep 03, 2008 12:40 am :
Doomguy87@Posted: Tue Oct 07, 2008 9:01 pm : thanks Brian i'll give this a try when i get the chance.

indianajonesilm@Posted: Wed Sep 22, 2004 2:40 am : Updated on October 3, 2004
Creating a simple cinematic with a moving camera.
Hello, this is my first tutorial. I'm a newbie, so if you find any mistakes please let me know.
Step 1.
Lets start by making a small room and add an "info_player_start" and a light.
Step 2.
Add a "func_cameraview".
Step 3.
Next make a NURB spline path for the camera to follow and name it "camera_spline".
Read Rayne's excellent NURBS tutorial here:
http://www.doom3world.org/phpbb2/viewtopic.php?t=5123
Instead of moving a light around the room, we will be moving the camera along this path.
Step 4.
Create a "func_mover" and name it "anchor". You must add a Key of cinematic" with a Value of "1". This is very important if you want the camera to move. I also add the Key "solid" with the Value of "0" so the camera can pass through stuff. You should also texture this func_mover with the "common\nodraw" texture.

Step 5.
Next we need to bind the func_camera view to the func_mover. Select the "func_cameraview" and add the Key "bind" with a Value of "anchor" in the entity inspectors window.
Step 6.
Add a "trigger_once" and give it a Key "call" with a Value of "camera_script". This is the trigger that will call the script we make in the next step.
Step 7.
That's it, now we need to write a script to switch the view to the func_cameraview and start it moving along the spline. Open up notepad and type the following:
Code:
void start_camera_spline()
{
$anchor.time(10); //How many seconds it will take to follow the spline from start to finish
$anchor.accelTime(1); //How long it takes to get up to speed
$anchor.decelTime(1); //How long it takes to decelerate
$anchor.startSpline($camera_spline); //Start the func_mover "anchor" moving along the spline
}
void camera_script()
{
sys.setCamera($func_cameraview_1); //Set the view to the camera
start_camera_spline(); //calls the script above
sys.wait(10); //The time to wait before switching view back to normal
sys.firstPerson(); //Turn off the camera and switch back to normal view
}
Step 8.
Save the script above as "your map name.script" and save it in the same folder as your map. For example if your map is called "cave.map" then name your script "cave.script".
Step 9.
Compile your map and start it up! As soon as you touch the "trigger_once" the view will switch to the camera and follow the spline path. If you wanted to make an intro cinematic before your map starts then either place the trigger on your info_player_start or just connect(Ctrl-K) your info_player_start with a "trigger_relay" that calls the script.
Step 10 (and other cool stuff).
Remember to add the
Key "cinematic" with the Value of "1" to all your func_mover's, func_rotator's, characters, and other entities that you want to see move in your cinematic.
Be sure to add the key to all lights that are triggered in your cinematic also. Any entity that is used during the cinematic, has to have this key set. (thanks Docgalaad). You can add special camera tricks to your cinematic by playing with the FOV (frame of view) to get cool warp effects. You can have many different cameras and just switch the view to another one whenever you want by triggering that camera with script or a trigger. If this tut has helped you out and you want to thank me, just mention me in the end credits of your map!


Here is an example of a simple cinematic which I made using the editor.

Download the pk4 here:
http://webpages.charter.net/aldanafx/stuff/cinematic_example.zipPlace the "cinematic.pk4" in your base folder and type "map cinematic" in the console to start it.
Update October 3, 2004How to aim the moving camera.(Thanks to rich_is_bored and MBolus for the help with the script)
Step 1.
First we need something for the camera to aim at, so we will add a "target_null" to the map. Place the target_null anywhere you want, and the camera will continuously aim at it while it travels along the spline. The target_null doesn't have to be in a fixed position, it can be binded to a mover and move along it's own spline if you wish.
Step 2.
Next we need to add a "target_setkeyval" to the map. A target_setkeyval is used to set or change Key's and Values of a targeted entity. In this case we will be using this target_setkeyval to add a Key\Val pair to the func_camera view. Now connect (Ctrl-K) the "target_setkeyval" to the "func_cameraview".
Step 3.
Select the "target_setkeyval" and give it a Key of "keyval" and a value of "target;target_null_1". It should look likethe following picture:

Step 4.
Now we need to add some additional script to tell the camera to continually aim at the target_null while it moves along the spline. Here is the previous script from above with the new aiming script added in:
Code:
void start_camera_spline()
{
$anchor.time(20);
$anchor.accelTime(1);
$anchor.decelTime(1);
$anchor.disableSplineAngles(); //You need to add this when aiming a moving camera.
$anchor.startSpline($camera_spline);
}
//This is the new aiming script:
void update_camera()
{
sys.threadname("aim_loop"); // Gives this thread the name "aim_loop" so it can be killed later.
while (1)
{
//This triggers the target_setkeyvall which in turn adds a key/val pair to the func_cameraview:
sys.trigger($target_setkeyval_1);
sys.waitFrame(); //This waits for the next frame before continuing
}
}
void camera_script()
{
sys.setCamera($func_cameraview_1);
start_camera_spline();
thread update_camera(); // This calls the function update_camera as a thread so it
// runs in the background.
sys.waitFor($anchor); // This halts execution until anchor finishes it's movement.
sys.killthread("aim_loop"); // This kills the thread "aim_loop"
sys.firstPerson(); //Turn off the camera and switch back to normal
}
Step 5.
As you see above, it is nessecary to use the "disableSplineAngles" command in your script when tracking something with the moving camera.
That's it, your camera should now aim at the target_null while it moves along the spline.
rich_is_bored@Posted: Wed Sep 22, 2004 3:06 am : Ah!
* slaps forehead *
I tried this exact thing before. The only difference was i didn't know about this cinematic key/value pair.
Thanks alot!

I'll try this out soon and verify if you have any corrections to make.
MBolus@Posted: Wed Sep 22, 2004 3:43 am : I have also been using this type of thing, including around areas such as hallways with teleporters, and found it to really enhance a level. Fov of 140 gives a pretty wide indoors view, compared with 90.
I would add a few minor points. There are a few additional useful keys. For example, you can specify whether an entity will hide after its anims, or whether it will stay to potentially attack, and there is a key you could use for the func_cameraview to indicate whether non-cinematic entities with enemies will be seen. Also, if you preferred you could use triggers to start and stop the new camera view.
rich_is_bored@Posted: Thu Sep 23, 2004 4:49 am : I've just tested it out and it works great.

Now the only thing left is to figure out a way to aim the camera at a target while it moves on the spline.
Great work though.

abigserve@Posted: Thu Sep 23, 2004 5:53 am : let us know how that turns out rich

Rayne@Posted: Thu Sep 23, 2004 7:32 am : Oh bè, you have to connect the func_cameraview's to a target_null (when you connect them, the cam view in the editor switch from the cameraview entity).. so you have only to bind and move even the target_null

rich_is_bored@Posted: Thu Sep 23, 2004 8:11 pm : Your right, targeting the camera_view to a target_null aims the camera.
The problem is that while the camera is initially aimed at the target_null when the map loads, it doesn't follow the null should the camera or null's position change.
indianajonesilm@Posted: Thu Sep 23, 2004 8:57 pm : Quote:
Now the only thing left is to figure out a way to aim the camera at a target while it moves on the spline.
I'm working on it.

I hope there is a way to do it. I added a little example of a cinematic for everyone. (you're in the credits

) I hope my free isp webspace doesn't exceed it's bandwidth limit.
rich_is_bored@Posted: Thu Sep 23, 2004 9:40 pm : That was impressive.

I hope you have the bandwidth to handle all the traffic you're going to get.
elroacho@Posted: Fri Sep 24, 2004 1:41 am : works like a charm...
a question tho, i want to pause the camera at the end of the spline for a few seconds while a big platform(func_door) moves down. i trigger the platform and the speakers for the sound of the moving plat a few seconds into the cinimatic. i can hear the souds of the plat but the func_door dosen't move until the cinimatic has completed and i can move again.
is their something special i have to do to trigger it to play in a cinimatic? if i trigger it with out the camera the func_door and the sound start at the same time like it's supposed to.
1 more thing, lets say you have a trigger to the cinimatic right before a door. then you put in a "recycling2_mancintro_player" to show the charector walking in the cinimatic. how to you make it so that when the cinimatic finishes, the player starts at a different spot then where they triggered it? like the player triggeres cinimatic from the beginning postition of "recycling2_mancintro_player" and when it finishes, i want the player to be at the end postition of "recycling2_mancintro_player".
help please, i'm tring real hard

indianajonesilm@Posted: Fri Sep 24, 2004 2:11 am : Quote:
is their something special i have to do to trigger it to play in a cinimatic?
You have to give the door a Key of "cinematic" with a Value of "1" in the entities inspector window in oder to see it move in your cinematic.
Quote:
how to you make it so that when the cinimatic finishes, the player starts at a different spot then where they triggered it?
Try using a "info_player_teleport". Make a Trigger in the start location. Ctrl-K the trigger with the info_player_teleport. When the player touches the tigger, (you can give it the Key "visualView" with the Value "Camera Name") it will switch views to that camera for your cinematic. (Check the usage of the different Key's in the entities inspector window). After the cinematic is over, your player will be in the location of the info_player_teleport.
That might work.
elroacho@Posted: Fri Sep 24, 2004 7:16 am : awesome, worked perfectly
thanx indianajonesilm
abigserve@Posted: Fri Sep 24, 2004 8:33 am : 
not working 4 me....i followed the tut exactly as well...
Oneofthe8devilz@Posted: Fri Sep 24, 2004 9:18 am : this stuff is amazing !!!!
I think this method combined with the animation export tool from "der_ton" are the ideal fundament for making professional machinima movies.
here is an example of what you can do with the tool
http://www.doom3world.org/phpbb2/viewtopic.php?t=5539
So, for the difficult parts like going up the stairs one could remove the stiffness of the models by creating an md5anim.......
Docgalaad@Posted: Sat Sep 25, 2004 2:47 am : OMG I LOST 6 HOURS TO DEBUG A FUNCTION
You have to be
EXTREMLY CAREFUL about the
cinematic key when you wish to move objects in front of a camera !
I didnt know this hidden key/value pair. And to learn how to use a camera, i looked into one used in the game. Unfortunatly, this one didnt make objects to move in the scenery.
I did a function moving one object, and activating a light to have a better view of what's happening. Once the cinematic switch back to first person view, i set the light off again.
My script was perfect until i test it. The script entered in a weird loop, and the camera made the script to never terminate. The game was stuck in the cinematic. I scanned every line and did a ton of modification. Until i look into THIS THREAD on the forum. Worse, i knew this post, i already read it 3 days ago. But i completly passed through the cinematic key issue.
So, if you have this trouble, yes you, i expect you will search for those keyword on the forum:
CAMERA FUNC_MOVER CINEMATIC LIGHT
Why light ? Cause the light on in my cinematic was a part of it, somehow, and i had to add the key/value cinematic/1 on the light, to get out of my cinematic and make the whole script running properly.
Any entity that is used during the cinematic, has to have this key set.
Not only the moving objects. I hope it's clear cause i'm a bit upset about the lost time


Cheers.
wheelbarrow@Posted: Sat Sep 25, 2004 3:02 am : I have a cinematic with 8 lights, 2 of them flashing, and 7 func_emitters, and they all work fine without the 'cinematic' key/value. The only thing with this key is an opening door....
Docgalaad@Posted: Sat Sep 25, 2004 3:04 am : I trust you. But removing the key on the light and my cinematic is stuck forever. The map works perfectly with the key set.
Do you trigger your lights and emitters during the cinematic ?
Cause i do on my part. Maybe...
xflame@Posted: Sun Sep 26, 2004 11:50 pm : I had a strange problem with this, when I ran my file, I got the error:
CANNOT BIND TO ITSELF!
wheelbarrow@Posted: Mon Sep 27, 2004 12:45 am : No, I don't trigger anything but the door during the cinematic. As for the error message above, just like it says, you've tried to bind something to itself. I did this myself by accident a while ago. Just go thru your entities, and make sure you're not binding the mover to itself. Easy mistake, easy to fix

indianajonesilm@Posted: Mon Sep 27, 2004 1:46 am : Quote:
abigserve: not working 4 me....i followed the tut exactly as well...
Sorry abigserve, I'm new to writing tutorials and I'm still figuring out this editor stuff. I've only been using it for a few weeks. I apologize for my inability to write a decent tutorial. Maybe I can help figure out the problem you're having if you give me a little more info. Did the view switch to the camera but didn't move? Did it switch to the camera view at all? Let me know.
Quote:
Oneofthe8devilz: here is an example of what you can do with the tool
Wow devilz that was awesome. The detail in the animation was unbelievable. There is no way a cinematic made in the editor can match what is possible in an md5anim. The possibilities are endless. I have a 3dsmax book, but it's the size of a phone book so I don't think I'll ever get around to learning it. I'll leave the md5anim's up to the pro's like you.

Quote:
Docgalaad: OMG I LOST 6 HOURS TO DEBUG A FUNCTION
I am so sorry Docgalaad! I should have worded it more clearly. Thank you for posting your solution. I hope you're not too mad at me.
